The Fetch API is a promise-based interface for fetching resources by making HTTP requests to servers from web browsers. It is similar to XMLHttpRequest
but better and more powerful.
In this tutorial, you will learn about the JavaScript Fetch API and how to use it to make asynchronous HTTP requests.
What is Fetch API
The Fetch API is a modern interface that allows you to make HTTP requests to servers from web browsers.
If you have worked with XMLHttpRequest (XHR) object, the Fetch API can perform all the tasks as the XHR object does. In addition, the Fetch API is much simpler and cleaner.
Also Read: Learn JavaScript Promises and Async/Await
It uses the Promise to deliver more flexible features to make requests to servers from the web browsers. The fetch()
method is available in the global scope that instructs the web browsers to send a request to a URL.
How to Use Fetch API ?
Using fetch API is really simple. Just pass the URL, the path to the resource you want to fetch, to fetch()
method.
fetch(url) .then(response => { // handle the response }) .catch(error => { // handle the error });
The fetch()
method returns a Promise, which can either be resolved or rejected.
- The promise resolves into a Response object. The Response object has a number of useful properties and methods to explore what we got from the request.
- The promise rejects if the
fetch()
method was unable to make the HTTP-request. Rejections can be due to network problems, or if no such URL exits.
We chain the fetch()
method with a then()
and catch()
method to enable us to use the response.
The then()
method is basically used to handle asynchronous tasks, such as fetching data from an API. So when we get a response from the fetch()
method, we will handle the response in then()
method.
If there is an error in fetching the request, for instance due to network problems or invalid URL, the catch()
method will handle this error.
In real life scenario a basic fetch request will look like this:
fetch('https://dummyjson.com/products/1') .then((response) => response.json()) .then((data) => console.log(data));
Output:
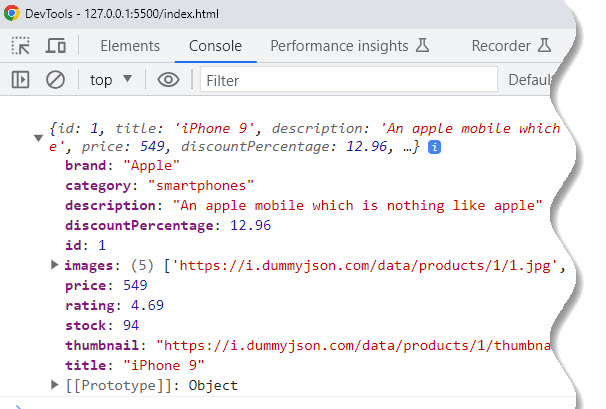
Reading the Response
The Response object we mention above represents the entire HTTP response, it does not directly contain the response body. To get the actual JSON body of the response, we use following methods:
response.json():
returns a Promise that resolves with the result of parsing as JSONresponse.text():
returns a Promise that resolves with a textresponse.formData():
returns a Promise that resolves with a FormDataresponse.error():
returns a new Response object associated with a network errorresponse.blob():
returns a Promise that resolves with a Blobresponse.arrayBuffer():
returns a promise that resolves with an ArrayBuffer
The Response object contains further data that needs to be converted into the required format in order to work with it.
There are several methods as we have mentioned above you can use to read the body of the response object. One of the popular is the json()
method.
The json()
method returns another Promise that decides the complete data of the fetched resource. We then access the data in another .then()
method
Example of fetching and reading data from an API
In this instance, we will fetch a single ecommerce product from the Fake Store API and then log the fetched data.
fetch('https://fakestoreapi.com/products/10') // view the complete content of the response .then((response) => response.json()) //access the actual data .then((data) => console.log(data)) //handle the error .catch(err => console.error(err));
Steps for fetching and reading data from an API
- Pass the
fetch()
method the required url to get the data from. In this instance, the URL will be https://fakestoreapi.com/products/10. It provides access to a single product. - Chain the
fetch()
method with thethen()
method. - If all goes well, in the
then()
method, we will receive a Response object from the server. - Extract the JSON body content from the Response object using the
json()
method. - The
json()
method will return a second Promise. This Promise will resolve to contain the fetched data by passing the data to anotherthen()
method. - In the
then()
method, we can now print the fetched data to the console or assign it to a variable. - If there is an error in fetching the data, the
catch()
method will capture the error, and we can log that error.
Output:
The output will be an object containing some dummy phone data- title, price, description etc.
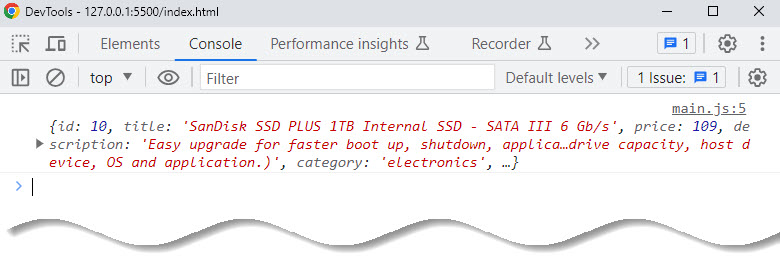
Instead of logging the output, you can assign this value to declared variable, and access the relevant data using the appropriate key:value pair.
In practice, you often use the async/await with the fetch()
method like this:
async function fetchProduct() { let response = await fetch('https://fakestoreapi.com/products/10'); let data = await response.json(); console.log(data); } fetchProduct();
Output will be same:
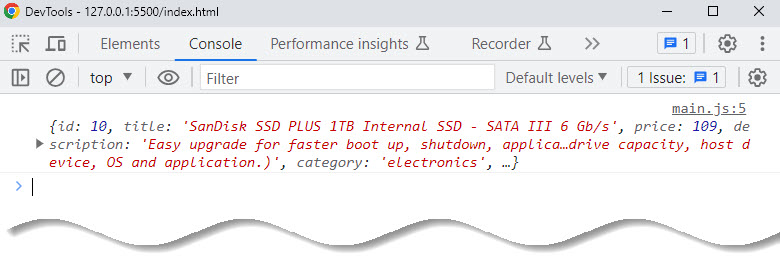
Handling the status codes of the Response
In the Response object, you can use the status
and statusText
properties to access the status code and status text, respectively.
When a request is successful, the status code is 200
and status text is OK
.
In the code below, we read the status code and status text from the server’s response:
fetch('https://fakestoreapi.com/products/10') .then(response => { // check the status code of the response object console.log(response.status) // an interpretation of the code console.log(response.statusText) // read the actual data from body of the response object return response.json() }) .then(data => console.log(data)) .catch(err => console.error(err));
Output:
200
OK
- A successful request will give a status code of
200
and a status text ofOK
. - If the requested URL throws a server error, the status code will be
500
. - If the requested resource does not exist, the status code will be
400
and status text will beNot found
.
JavaScript Fetch isn’t Just for GETting
By default, the Fetch API uses GET
method for asynchronous requests. But with fetch method you can do more than just get data from the server.
You can post, delete, and update data to the server. In order to do so, you need to add a second parameter to the fetch method and use an HTTP request method.
HTTP Request Type:
Method | Usage | |
GET | To get data | |
POST | To send new data | |
PUT | To update existing data | |
DELETE | To delete item from data |
GET, POST, PUT, DELETE Request
In general the Fetch API is able to retrieve data from any URI. This can be just a local file or a remote server. For the following examples we will be using the JSONPlaceholder service.
It is exposing multiple endpoints which can be used for sending GET or POST requests to. In the following you can see the list of resources available:
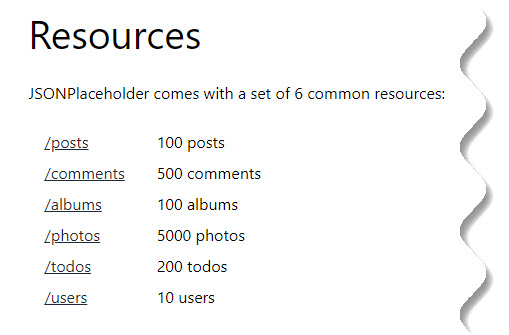
GET Request
For this example, we will use the /users endpoint. First let’s start by adding the some HTML code to the body section of the HTML file:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Fetch API</title> </head> <body> <div> <h1>Getting Started With Fetch API</h1> <button id="fetchUserDataBtn">Fetch User Data</button> </div> <hr> <div id="response"></div> <script src="main.js"></script> </body> </html>
A button is added with id
equal to fetchUserDataBtn
. Furthermore a div element with id
response
is added.
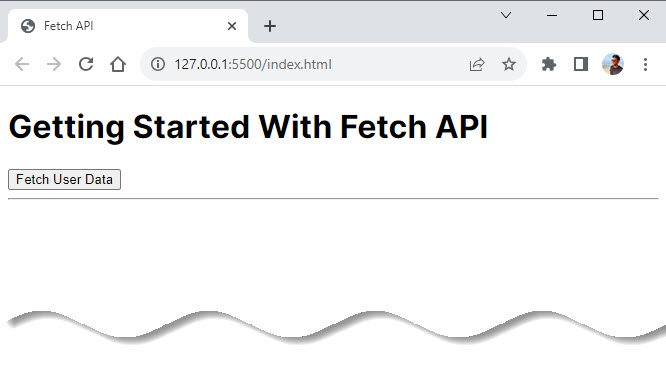
Now we are ready to further complete the implementation by adding a script section:
document.getElementById('fetchUserDataBtn').addEventListener('click', fetchUserData); function fetchUserData() { fetch('https://jsonplaceholder.typicode.com/users/1') .then(response => response.json()) .then(json => console.log(json)) }
This JavaScript code is used to first add a click event listener function to the fetchUserDataBtn
. The function contains the code which is needed to retrieve data from endpoint https://jsonplaceholder.typicode.com/users/1. This endpoint is returning the user data object with ID 1 in JSON format.
Also Read: Learn AddEventListener in JavaScript
The endpoint is passed into the call of the fetch function as string. The call of fetch returns a promise. This means that we are able to attach calls of the then
method in order to wait for the promise to be resolved.
The first call of then
is used to execute an arrow function to extract the JSON part of the response object. The second call of then
is used to output the JSON result to the console.
In the following screenshot you can see the output in the browser console after clicking on the button:
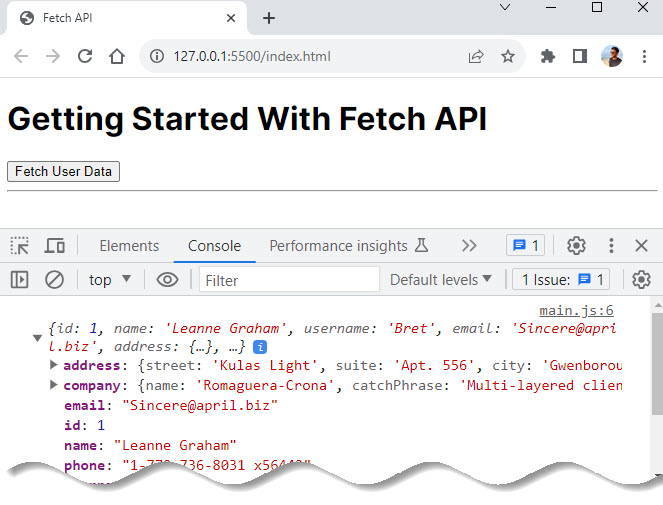
Let’s adapt the code a little bit. Instead of requesting only one user data objects lets retrieve all user objects by using endpoint https://jsonplaceholder.typicode.com/users/:
document.getElementById('fetchUserDataBtn').addEventListener('click', fetchUserData); function fetchUserData() { fetch('https://jsonplaceholder.typicode.com/users/') .then(response => response.json()) .then(json => console.log(json)) }
Again the output is written to the console, so that you should be able to see a list of user objects like in the following screenshot:
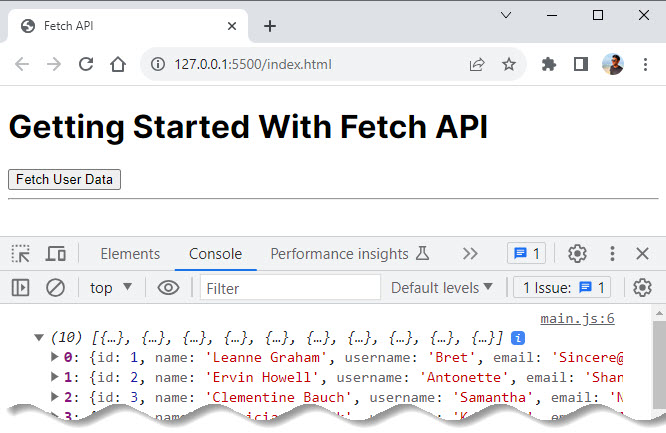
We have already added a div
element with id
response
to the HTML code. Let’s use this div
element to output the list of user names directly on the website:
document.getElementById('fetchUserDataBtn').addEventListener('click', fetchUserData); function fetchUserData() { fetch('https://jsonplaceholder.typicode.com/users/') .then(response => response.json()) .then(users => { let output = '<h2>Lists of Users</h2>'; output += '<ul>'; users.forEach(function (user) { output += ` <li> ${user.name} </li> `; }); output += '</ul>' document.getElementById("response").innerHTML = output; }); }
The HTML code which is used to output the list of user names is stored in variable output
. The forEach
method is used to loop over the items available in the users
array.
A function is passed into the call of forEach
. This function is executed for every item in the array. The current user item is passed into this function via parameter, so that you have access to the user properties from within this function.
The list item <li>...</li>
element is added to output
by using a template string (back-ticks are used). Template strings can contain placeholders. These are indicated by the dollar sign and curly braces (${user.name}
).
By using this placeholder we are able to add the name value directly to the HTML output.
Finally the HTML code which is stored in the variable output is added to the website by using the following line of code:
document.getElementById("response").innerHTML = output;
The output in the browser should then look like what you can see in the following:
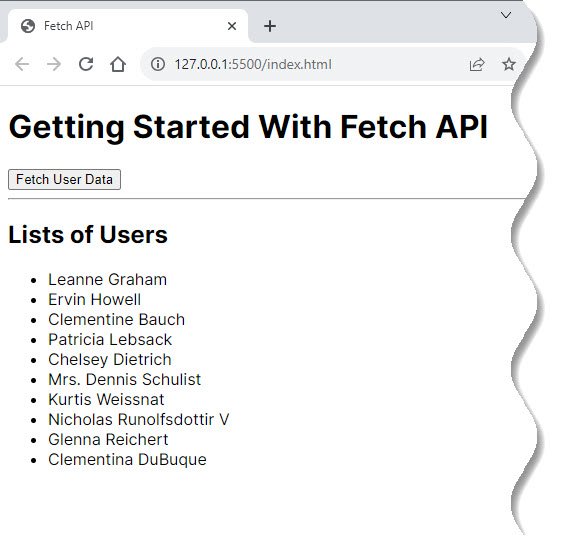
POST Request
In the following let’s take a look at another example which is sending HTTP POST requests via the Fetch API. Let’s take a look at the list of available endpoints at jsonplaceholder.
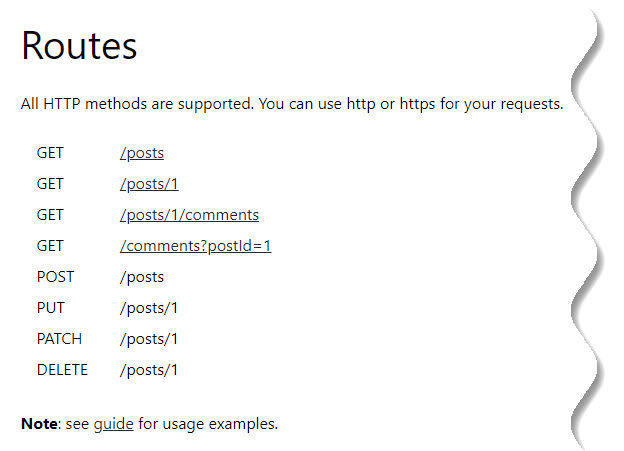
As you can see there is one endpoint (/posts) available which is of type POST. This endpoint is accepting new post entries in JSON format consisting of the properties title
and body
.
Let’s add a simple form to our application which consists of two input fields so that the user is able to provide values for a new post entry:
<form id="addPostForm"> <div> <input type="text" id="title" placeholder="Title"> </div> <div> <textarea id="body" placeholder="Body"></textarea> </div> <input type="submit" value="Submit"> </form>
Furthermore a submit button is part of the form. To handle the submit event we need to add a new event handler function next:
document.getElementById('addPostForm').addEventListener('submit', addPost);
This line of code is attaching the addPost
function as an event handler to the submit event of the form. The implementation of the addPost
function consists of the following code:
function addPost(event) { event.preventDefault(); let title = document.getElementById('title').value; let body = document.getElementById('body').value; const myPost = { title: title, body: body }; fetch('https://jsonplaceholder.typicode.com/posts', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify(myPost) }) .then((res) => res.json()) .then((data) => console.log(data)) }
The first thing which needs to be done inside of addPost
is to call the event.preventDefault()
method to prevent the default submit behaviour of the browser.
This enables us to implement our own submit logic in the following lines of code.
Before sending the HTTP POST request to the endpoint by using the Fetch API we need to prepare the new user object based on what has been entered in the from by the user.
The values which have been entered in the input elements are stored in the variables title
and body
and are used to create a new user object which is stored in myPost
.
The HTTP POST request is sent by calling the fetch function. Two parameters are passed into this function call:
- The URI of the endpoint as string
- A configuration object
The configuration object needs to contain the method property which needs to be set to the string POST. Furthermore we need to add the headers property and the body property.
The value which is assigned to headers is an object container properties which are added to the request header. Here we are setting the Content-Type header property to the string value application/json.
Furthermore we need to add the body property and set the value to the JSON string representation of the new post object.
This is done by calling the JSON.stringify
method and passing in the myPost
variable as a parameter.
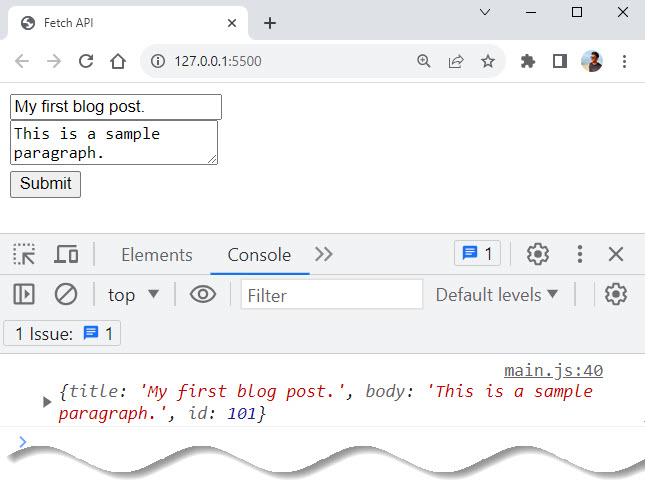
PUT
and DELETE
Request we will cover in upcoming articles to limit the length of this article.
Conclusion
That’s all devs, go ahead and try fetching some data from your favorite APIs.
In summary JavaScript Fetch API is a huge improvement over XMLHttpRequest
with an easy-to-use interface and works great for fetching resources.
We hope you enjoyed the tutorial, Thanks For Reading.
Resource
- JavaScript Fetch API – JavaScript Tutorial
- JavaScript Fetch example – Dev.to
- Fetch API in JS – Medium
- JS Fetch Post and Header Examples
Add comment