To start using React you don’t have to learn a lot. Learn the concept of components, state
, props
, and hooks — and you know how to use React.
In this blog, we will learn what are props in react and how we can use them in our application to use dynamic data. We will also learn about props syntax, the different ways to pass props and the setting up of the default value of props.
What are Props in React?
React components use props to communicate with each other. Every parent component can pass some information to its child components by giving them props.
Props are basically used to pass data between React components.
So props
, short for “properties”, is used for passing data from one component to another. However, the data being passed is uni-directional, meaning it can only be passed from the parent to the child.
Also kindly remember, React props can not be modified in a component.
The concept of props builds on components. So we can’t successfully work with props without having a component to work with.
Let’s build and connect the components that we will be working with in this tutorial together.
Below is the structure of the parent component:
App.jsx
import Product from './Product'; function App() { return ( <> <h1>PRODUCTS</h1> <Product /> </> ); } export default App;
Below is the structure of the child component:
Product.jsx
import React from 'react' const Product = () => { return ( <div> <img src="https://i.ibb.co/c3QdTYb/sample-shoe.jpg" alt="sneakers" /> <h4>Cyxus</h4> <p>Non-Slip Fitness Leisure Running Sneakers</p> <h4>$29</h4> </div> ) } export default Product
Our goal is to be able to display all the different products which vary in name, price, appearance, and description to users.
Of course, we can reuse the product component as many times as we want by just re-rendering the component.
For example:
App.jsx
import Product from './Product'; function App() { return ( <> <h1 style={{ textAlign: 'center' }}>PRODUCTS</h1> <div style={{ display: 'flex', justifyContent: 'space-around' }}> <Product /> <Product /> <Product /> </div> </> ); } export default App;
Output:

We can see the React functionality of repeating a particular design without writing much code in play here.
But we haven’t yet achieved our goal, right? While we want to reuse the component, we also want to update the name of the product, the price, the description, and the image without having to hard code any data in the Product.jsx component.
This is where we can use props in React to make our data output dynamic.
How to Use Props in React?
Before we go deeper, it is important to note that React uses a one-way data flow. This means that data can only be transferred from the parent component to the child components.
Also, all the data passed from the parent can’t be changed by the child component.
This means that our data will be passed from App.jsx which is the parent component to Product.jsx which is the child component (and never the other way).
How to Send Props Into a Component
How props are passed into a component is similar to how attributes work in HTML elements.
For example, when you want to pass attributes into an input element in HTML, you will write the attribute and attach the value of the attribute to it, just like this:
<input type="text" placeholder="Cyxus" />
Also, when sending props (which are also properties and can be likened to attributes), you attach your values to them.
Below is the syntax:
<ComponentName property1="value" property2="value" property3="value" />
Within the component tag, after writing the component name we will assign a value to each property.
Now let’s use the syntax above to pass data into the App.jsx component:
<Product img="https://i.ibb.co/c3QdTYb/sample-shoe.jpg" name="Cyxus" desc="Non-Slip Fitness Leisure Running Sneakers" price="$29" />
In the code above, the component name within the tag is Product, and the first property or prop is img with its value attached to it. Then we have name which is the second property and desc which is the third property finally we have price (these are also assigned values).
When we structure the App.jsx component properly, it will now look like this:
App.jsx
import Product from './Product'; function App() { return ( <> <h1 style={{ textAlign: 'center' }}>PRODUCTS</h1> <div style={{ display: 'flex', justifyContent: 'space-around' }}> <Product img="https://i.ibb.co/c3QdTYb/sample-shoe.jpg" name="Cyxus" desc="Non-Slip Fitness Leisure Running Sneakers" price="$29" /> </div> </> ); } export default App;
There is a slight difference between writing HTML attributes and passing in props: while HTML attributes are special keywords already provided for you, you customize and define props in React.
For example, we created the properties; ‘img‘, ‘name‘, ‘desc‘, and ‘price‘ above. Then, we attached the values of the props alongside them.
How to Access and Use Props in React
The component receives props as a function parameter. It uses the value of props by defining the parameter as props objects.
Below is the syntax:
//the function receives 'props' as a parameter function function Product(props) { return ( <div> //it uses the value of props by defining //the parameter as props objects <img src={props.objectName} alt="products" /> <h4>{props.objectName}</h4> <p>{props.objectName}</p> <h4>{props.objectName}</h4> </div> ); } export default Product
Let’s relate the syntax above to our Product.jsx by receiving props as a parameter function and by defining props as object:
Product.jsx
import React from 'react'; // the function receives 'props' as a parameter function const Product = (props) => { return ( <div> {/* it uses the value of props by defining the parameter as props objects */} <img src={props.img} alt="products" /> <h4>{props.name}</h4> <p>{props.description}</p> <h4>{props.price}</h4> </div> ); }; export default Product;
We have successfully made the data we passed into the Product.jsx component dynamic.
To reuse the ‘Product.jsx’ component to show the data of other products, all we need to do is to attach the new data or values when re-rendering the component.
Below is the syntax for this:
<ComponentName property1="valueA" property2="valueB" property3="valueC" /> <ComponentName property1="valueD" property2="valueE" property3="valueF" /> <ComponentName property1="valueG" property2="valueH" property3="valueI" />
Now let’s relate the syntax above to our App.jsx:
App.jsx
import Product from './Product'; function App() { return ( <> <h1 style={{ textAlign: 'center' }}>PRODUCTS</h1> <div style={{ display: 'flex', justifyContent: 'space-around' }}> <Product img="https://i.ibb.co/c3QdTYb/sample-shoe.jpg" name="Cyxus" desc="Non-Slip Fitness Leisure Running Sneakers" price="$29" /> <Product img="https://i.ibb.co/p0S29Zf/sample-shoe-1.jpg" name="Vitike" desc="Latest Men Sneakers -Black" price="$100" /> <Product img="https://i.ibb.co/1Zy1j34/sample-shoe-2.jpg" name="Aomei" desc="Men's Trend Casual Sports Shoe" price="$40" /> </div> </> ); } export default App;
The live output of the code above now displays image, name, description and price of the each product.
Product.jsx
import React from 'react'; // the function receives 'props' as a parameter function const Product = (props) => { return ( <div> {/* it uses the value of props by defining the parameter as props objects */} <img src={props.img} alt="products" /> <h4>{props.name}</h4> <p>{props.description}</p> <h4>{props.price}</h4> </div> ); }; export default Product;
Here is the live output:

Destructuring Props in React
Now that we have achieved the functionality we aimed for, let’s format our Product.jsx by making use of destructuring.
This is a feature of JavaScript that involves assigning pieces of data from an object or array to a separate variable so that the variable can hold the data coming from the array or object.
Also Read: Master Destructuring Assignment (Array & Object)
Props are objects. So to destructure objects in React, the first step is to group your properties within a set of curly braces.
Then you can either store it into a variable called props within the body of the function or pass it directly as the function’s parameter.
The second step is to receive the properties where you need them by stating the names of the properties without attaching the prefix ‘props’.
Below is an example of the two ways you can destructure in React:
Product.jsx
import React from 'react'; const Product = (props) => { // First Step: Destructuring within the body of the function const { img, name, description, price} = props ; return ( <div> <img src={img} alt="products" /> {/* Second Step: receive the properties where you need them by stating the names of the properties without attaching the prefix 'props.' */} <h4>{name}</h4> <p>{description}</p> <h4>{price}</h4> </div> ); }; export default Product;
OR
Product.jsx
import React from 'react'; //First Step: Destructuring within function's parameter const Product = ({ img, name, description, price}) => { return ( <div> <img src={img} alt="products" /> {/* Second Step: receive the properties where you need them by stating the names of the properties without attaching the prefix 'props.' */} <h4>{name}</h4> <p>{description}</p> <h4>{price}</h4> </div> ); }; export default Product;
Destructuring in React makes our code more readable and more concise. Note that the two ways of destructuring that we demonstrated above will always have the same output.
Output:

Values of Props
In most of the previous examples, the values of props were strings. But often you would like to set props to values like numbers, booleans, objects, arrays, and even variables.
React doesn’t put any restriction on what value a prop can have. But all values, except double-quoted string literals, have to be wrapped in curly braces prop={value}
.
Use the following guide to set different types of values on your components.
Example of String literals:
<MyComponent prop="My String Value">
Example of template literals with variables:
<MyComponent prop={`My String Value ${myVariable}`}>
Example of Number literals:
<MyComponent prop={42} />
Example of Boolean literals:
<MyComponent prop={false} />
Example of Plain object literals:
<MyComponent prop={{ property: 'Value' }} />
Example of Array literals:
<MyComponent prop={['Item 1', 'Item 2']} />
Example of passing JSX:
<MyComponent prop={<Message name="Ram" />} />
Variables having any kind of value:
<MyComponent prop={myVariable} />
Specifying a default value for a prop
Sometimes, we don’t want the props value to be empty or when no value is specified, at that time we can specify a default value just by destructuring and putting equal sign and the default value right after the parameter.
For example, let’s make the name prop inside the <Greet/> component optional, but default to ‘Admin’.
App.jsx (parent component)
import Greet from './Greet'; function App() { return ( <> <Greet/> </> ); } export default App;
Greet.jsx (Child Component)
import React from 'react'; const Greet = ({ name = 'Admin' }) => { return ( <> <h1>Hello {name}</h1> </> ); }; export default Greet;
Looking at the parameter destructuring { name = ‘Admin’ } you would notice that if name prop is not indicated, then it defaults to ‘Admin’
value. Great!
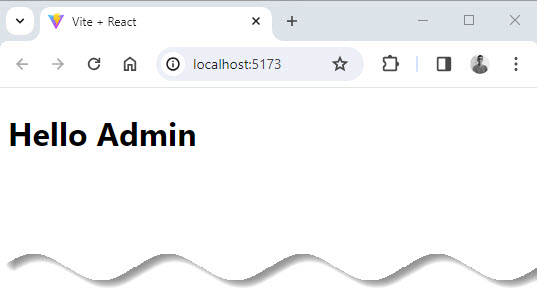
However, if indicated, name accepts the actual value:
App.jsx (parent component)
import Greet from './Greet'; function App() { return ( <> <Greet name='Sunil'/> </> ); } export default App;
Greet.jsx (Child Component)
import React from 'react'; const Greet = ({ name = 'Admin' }) => { return ( <> <h1>Hello {name}</h1> </> ); }; export default Greet;
Output:
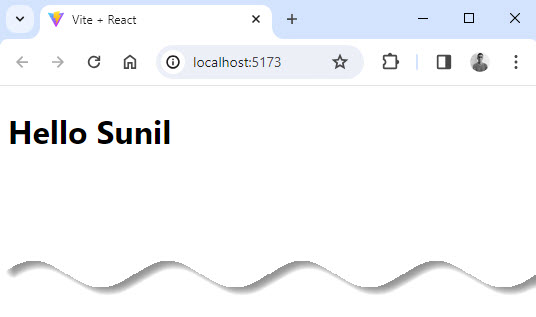
Forwarding props with the JSX spread syntax
What if we have an object whose properties we want to pass down as individual prop values?
This object has a lot of properties, however. Do we need to manually create individual props and set the prop to object.propertyName?
No – instead of doing that for every property, we can very easily take the object and spread its properties down to a component as individual prop values using the object spread operator {…myPropObject}.
Also Read: JavaScript Spread Operator (…)
App.jsx
import Myblog from './Myblog'; function App() { const data = { title: 'my title', description: 'my description', button: 'see more', }; return ( <> <Myblog {...data} /> </> ); } export default App;
Myblog.jsx
import React from 'react' const Myblog = ({title, description, button}) => { return ( <div> <h1>{title}</h1> <p>{description}</p> <button>{button}</button> </div> ) } export default Myblog
Output:
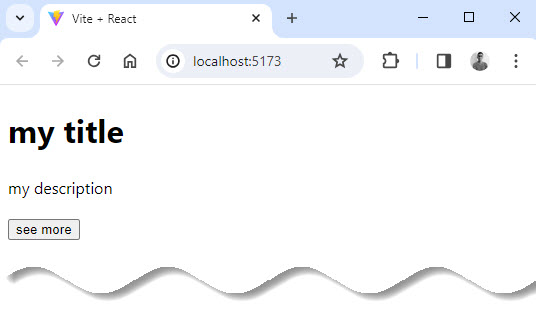
Conclusion
You have made it till the end of this blog 🤗. More such blogs are on the line .
In this blog, we learned about props in react, how they are used, their syntax, and different ways of using props. Also we learned props are the data passed in from the parent component. They are immutable inside the component that receives them.
It would be encouraging if a small comment would be there on the blog. we go through each one of them so do comment 😉.
Keep coding, keep rocking 🚀
Add comment