React is an efficient and flexible JavaScript library for building user interfaces. It breaks down complex UIs into small, isolated code called “components”. By using these components, React only concerns itself with what you see on the front page of a website.
What are React Components?
Components are the heart of any React Application. You can think of components as building blocks. You can make a beautiful structure using these building blocks.
The same thing applies to components in React. It’s a piece of UI. You can make a whole UI merging these components.
You can think of them as custom HTML elements. They are independent and isolated from each other and because of that, they can be maintained and managed very easily. But the most important thing is, they are reusable.
Components are like JavaScript functions that return HTML elements.
So, we can create a React component once and use it as many times as you want. That gives the flexibility to React applications.
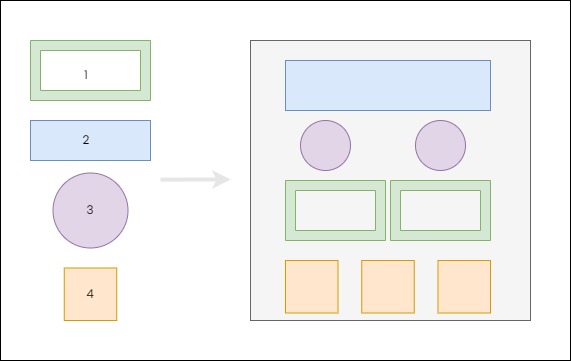
Above image would be an easy way to summarize the usage of components. The image has a typical webpage like layout where it has a hero section with services section displayed in 2 columns and three blog posts listed at the bottom.
Without using React we would be writing the HTML for all the 2 services section and 3 blog posts which doesn’t follow DRY principle.
By using component concept, we can write one services section, for instance, in services.js
and reuse it in second column by just declaring the component name, which will save time and easy to maintain.
So, components are independent and reusable bits of code. They serve the same purpose as JavaScript functions, but work in isolation and return HTML.
In React, we have two main types of components:
- Class components
- Functional components
Class components
Class components are ES6 classes. It starts with the class
keyword followed by the name starting with an uppercase letter. It also extends React.Component
class that gives the created component access to its functions.
Class components optionally receive props
as input and renders the JSX. It can also maintain the private internal state
of a particular component.
Class component should always start with capital letter and the props should never be modified.
Also it must have render()
method and must return a React element.
Let’s see an example of react class component:
Welcome.js
import React, { Component } from 'react' class Welcome extends Component { render() { return <h1>Welcome {this.props.name}</h1> } } export default Welcome // {this.props.name} => Return value // (props) => argument // Welcome => Function name
App.js
// import './App.css'; import Welcome from './components/Welcome'; function App() { return ( <div> <Welcome name="Sunil"/> </div > ); } export default App; //Welcome => call a function // name="Sunil" => pass arguments
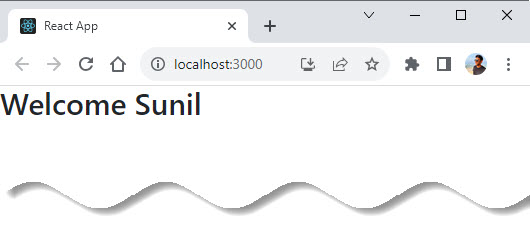
❎Drawbacks of Class Components
- Class components are slight slower than their functional counterparts. The performance difference increases when the number of components in the app increases.
- Class components involve a lot more coding on the programmer’s side making them slightly more inefficient to use.
Functional Components
Functional components as the name describes are functions in JavaScript. They may or may not take arguments called props
and they return React elements that are rendered in a browser.
Let’s see an example of react functional component:
Greet.js
import React from 'react' function Greet(props) { return <h1> Hey, {props.name} </h1>; } export default Greet // {props.name} => Return value // (props) => argument // Greet => Function name
App.js
import Greet from './components/Greet'; function App() { return ( <div> <Greet name="Sunil" /> </div > ); } export default App; //Greet => call a function // name="Sunil" => pass arguments
Output:
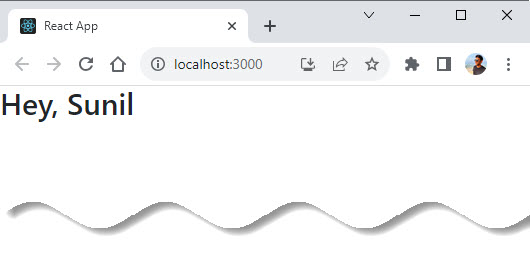
Kindly remember, you should never modify props
. You should use functional components when your component simply receives props
and render something that does not have its State(React < 16.8).
In React 16.8, Functional components can hold state using React hooks. It’s a good practice to use functional components over class components.
✅Advantages of Functional Components
Functional components has following advantages:
- Easy to understand syntax
- Easy to write unit tests
- Less code to write
- You can use the
useState
anduseEffect
hook v16.8
Differences between Functional Components and Class Components
The most obvious one difference is the syntax. A functional component is just a plain JavaScript function which accepts props
as an argument and returns a React element.
A class component requires you to extend from React.Component
and create a render function which returns a React element.
Conclusion
React components empower developers to build scalable and maintainable web applications. With their reusability and modularity, React components streamline the development process.
React components also provide their own logic and a layer of separation in general so that one can add and update logic without causing a lot of issues.
Hence, React components continue to revolutionize web development, providing an efficient and powerful toolset for building modern applications.
Add comment