This article unravels the mystery behind the “unique key” warning message often appearing in React projects. It discusses its importance and demonstrates how to add and create unique keys effectively!
What are React Keys?
When working with any type of list in React, you will often encounter this warning if you forgot to include a key prop:
Warning: Each child in a list should have a unique key prop β.
So, why does React tell you to include unique key prop and why is it important?
Simply put, they are props that are passed in child elements of list in order to:
- identify which elements are added
- identify which elements are updated
- identify which elements are removed
Hence, keys serve as identification for an element just like how passports are used to identify people.
π£ Also Read: How to Render Lists in React using map Method
Why Do We Need It?
At this point, you may wonder why do we need it? After all, we could identify elements by their id, className, parent/child, index, props, etc.
The answer is because of React’s Diffing Algorithm.
Diffing Algorithm: A Brief Explanation
A React app is made up of a tree of components. Whenever there’s a prop or state change in any component, React re-renders its components into its virtual DOM.
π£ Also Read: React Virtual DOM β Teach Me Like a Kid
The diffing algorithm compares the new virtual DOM with the old DOM at each level of the component tree, starting from the root node.
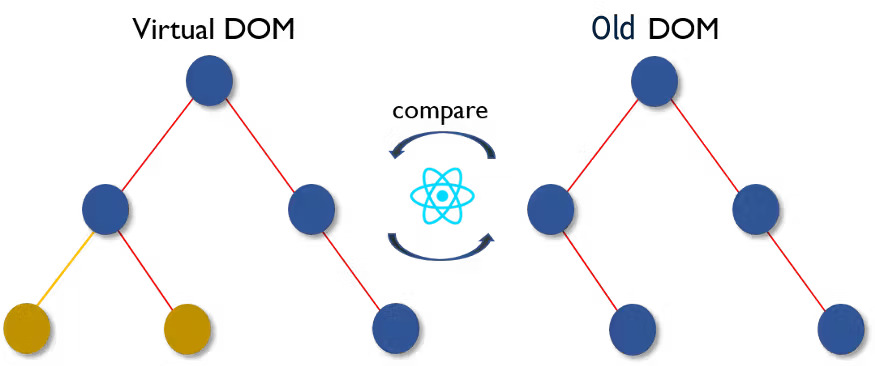
The algorithm finds the minimum number of operations required to update the real DOM.
This is how it does it:
A. Compare node by types (i.e. <div> vs <span>)
If different, destroy and build a new component from scratch.
// virtual DOM
<div><MyComponent/></div>
// real DOM
<span><MyComponent/></span>
E.g. This results in <MyComponent/>
being destroyed and re-built.
B. If nodes have same type, compare by attributes.
If different, only update the attributes.
// virtual DOM
<div className="after" title="stuff" />
// real DOM
<div className="before" title="stuff" />
E.g. This results in an update of className
to after
.
C. What about lists?
For lists, React will recurse on both their children simultaneously, find any differences, then patch them to the real DOM if there are any.
// virtual DOM
<ul>
<li>first</li>
<li>second</li>
<li>third</li>
</ul>
// real DOM
<ul>
<li>first</li>
<li>second</li>
</ul>
E.g. This results in the <li>third</li>
being added after <li>second</li>
.
So far so good? But now, instead of adding an element at the bottom of the list, what if we add a new element at the beginning?
// virtual DOM
<ul>
<li>zero</li>
<li>first</li>
<li>second</li>
</ul>
// real DOM
<ul>
<li>first</li>
<li>second</li>
</ul>
This example will result in React re-rendering every single <li>
to the real DOM because it doesn’t realize that it can simply add <li>zero</li>
to the beginning of the list.
This inefficiency can cause problems, especially in larger apps. Hence, keys provide a simple solution to this issue.
How to Add and Create Unique Keys
Now, let us see how we create a list in React. Below is an array you will be working with.
It contains info about applicants to a mentorship workshop. The array is stored in applicants variable.
const applicants = [
{
id: '0',
name: 'Sunil',
work: 'Freelance Developer',
blogs: '54',
websites: '32',
hackathons: 'none',
location: 'India',
},
{
id: '1',
name: 'Anil',
work: 'Fullstack Developer',
blogs: '34',
websites: '12',
hackathons: '6',
location: 'India',
},
{
id: '2',
name: 'Gopal',
work: 'Web Developer',
blogs: '28',
websites: '8',
hackathons: '3',
location: 'India',
},
];
Now, you need to return JSX that renders every applicant name as presented from the array.
To get the names of the applicants, you can easily do that with JavaScriptβs map()
method. Below is how you can map every applicantβs name:
Applicants.jsx
import React from 'react'; const Applicants = () => { const applicants = [ { id: '0', name: 'Sunil', work: 'Freelance Developer', blogs: '54', websites: '32', hackathons: 'none', location: 'India', }, { id: '1', name: 'Anil', work: 'Fullstack Developer', blogs: '34', websites: '12', hackathons: '6', location: 'India', }, { id: '2', name: 'Gopal', work: 'Web Developer', blogs: '28', websites: '8', hackathons: '3', location: 'India', }, ]; return ( <div> <ul> {applicants.map(function (data) { return <li key={data.id}>Applicant Name: {data.name}</li>; })} </ul> </div> ); }; export default Applicants;
App.jsx
import Applicants from './Applicants'; function App() { return ( <> <Applicants /> </> ); } export default App;
This is the expected code output:
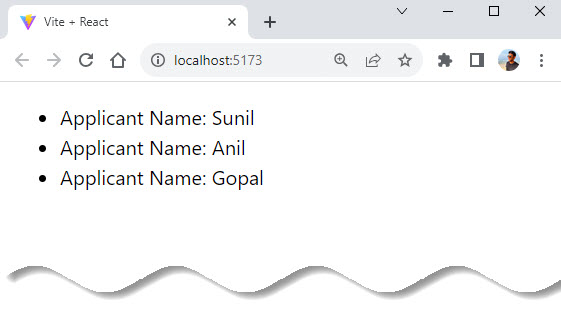
If you do not have a unique key ID in your data then the recommended way of creating a unique key is by UUID library , which can be easily installed in your projects using the Node Package Manager.
The UUID quickstart provides the following instructions:
Step 1: Install
npm install uuid
Step 2: Create a UUID
import { v4 as uuidv4 } from 'uuid';
uuidv4(); // β¨ '9b1deb4d-3b7d-4bad-9bdd-2b0d7b3dcb6d'
Here is an example of how to set UUID key values to list items in a React project:
Applicants.jsx
import React from 'react'; import { v4 as uuidv4 } from 'uuid'; const Applicants = () => { const applicants = [ { name: 'Sunil', work: 'Freelance Developer', blogs: '54', websites: '32', hackathons: 'none', location: 'India', }, { name: 'Anil', work: 'Fullstack Developera', blogs: '34', websites: '12', hackathons: '6', location: 'India', }, { name: 'Gopal', work: 'Web Developer', blogs: '28', websites: '8', hackathons: '3', location: 'India', }, ]; return ( <div> <ul> {applicants.map(function (data) { return <li key={uuidv4()}>Applicant Name: {data.name}</li>; })} </ul> </div> ); }; export default Applicants;
App.jsx
import Applicants from './Applicants'; function App() { return ( <> <Applicants /> </> ); } export default App;
This is the expected code output:
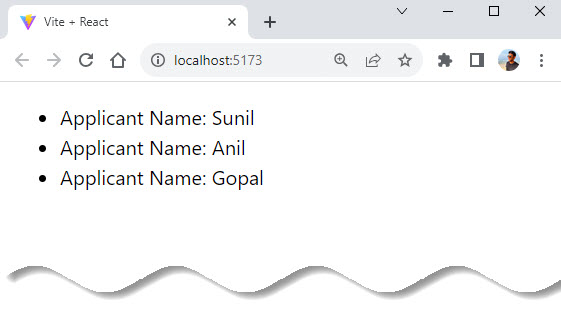
Best Ways to Use Keys in React
Follow these best practices for using keys in React to get the most out of them:
Tip 1: Each item in the list should have its key
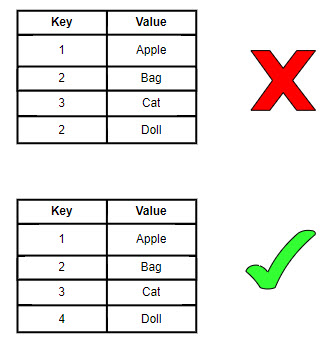
Tip 2: The keys should stay the same over time
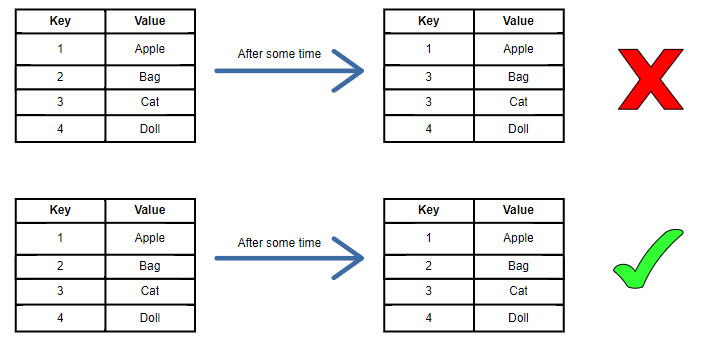
Tip 3: Keys should be steady, meaning they shouldn’t change when the list’s order changes
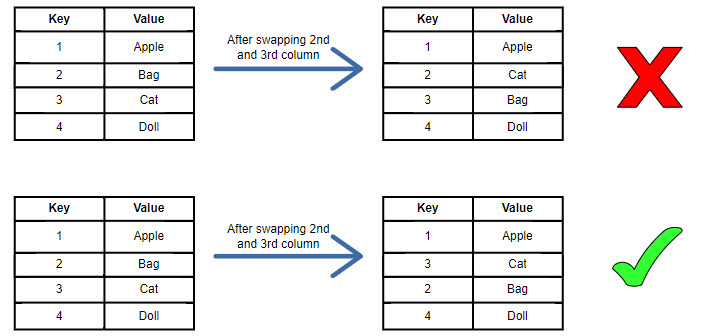
Tip 4: Keys should be added to the list’s top-level elements, not child elements
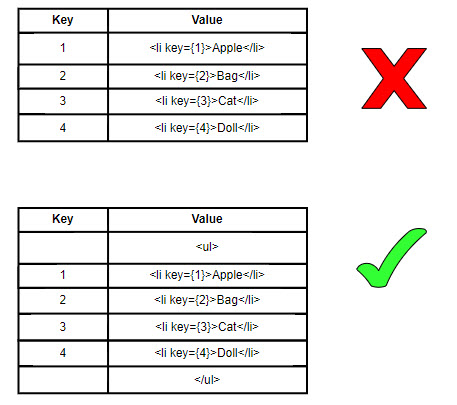
In the wrong example, the keys are added to the child elements (<li>
) instead of the top-level element (<ul>
).
This violates the best practice of adding keys to the top-level elements in the list. Whereas, in the right example, the key is added to the top-level element (<ul>
) instead of the child elements (<li>
).
This follows the best practice of adding keys to the top-level elements in the list.
Conclusion
Assigning keys to list elements is essential for maintaining the performance and stability of React applications.
Unique keys in React can be assigned manually, chosen from an array, generated using a library like UUID, or created by combining existing data with a unique identifier.
By understanding the importance of unique keys and implementing them appropriately, you can create more reliable and responsive user interfaces for your applications.
Add comment